Favorites
Customize ESPN
ESPN Sites
ESPN Apps
Passan: Juan Soto's Mets deal transforms New York -- and the sport
In choosing the Mets, Soto flipped the script in New York -- and across the sport.
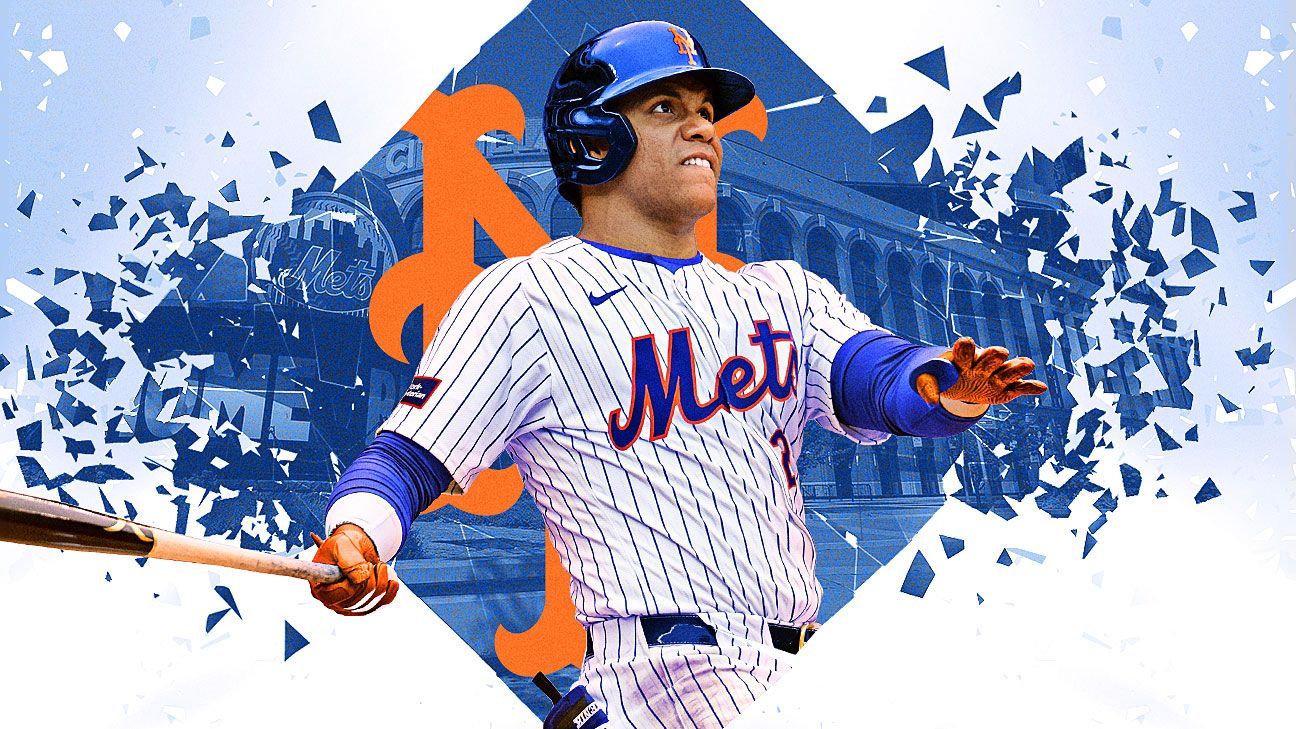
What Soto's $765 million deal means for the Mets -- and the rest of MLB
We debate the fallout of a mind-boggling contract.
TOP HEADLINES
Chiefs clinch AFC West title after game-winning FG doinks off crossbar

THE BRACKET IS SET
COLLEGE FOOTBALL PLAYOFF
National title paths for all 12 CFP teams: Here's how each could get hot and win it all
The College Football Playoff bracket is out. Here's the road map for each team to win the national championship.
First look at all four first-round games: X factors, key players and more
How all eight teams can win their first-round matchups.
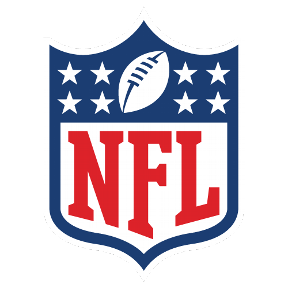
BREAKING DOWN A BUSY SUNDAY
Potential overreactions to NFL Week 14: Making sense of five QB situations and one dangerous NFC team
Minnesota was smart to move on from Cousins? But Seattle and Carolina need to stick with their QBs?
What we learned: We answered big questions and sized up every matchup
NFL Nation reporters react to all the action, answering the biggest questions coming out of each game.
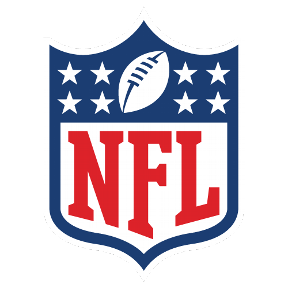
NFL SCOREBOARD
SUNDAY'S GAMES
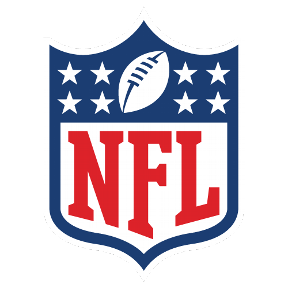
TOP PERFORMANCES OF THE DAY
Sam Darnold tosses five TD passes in Vikings' win
Puka Nacua makes a jaw-dropping catch, ends night with two TDs
Ashlyn Watkins rocks rim with one-handed dunk in win over TCU

WHO WILL WIN THE CFP? 🏆
FIRST ROUND BEGINS DEC. 20
Predicting the entire College Football Playoff -- and all 36 bowls: Score picks for each game
We made predictions on the final score of every bowl matchup. Check back to see how we fared.
College Football Playoff predictions: Picking every game in every round
Our college football writers predict the outcomes of each CFP matchup and choose their champion.
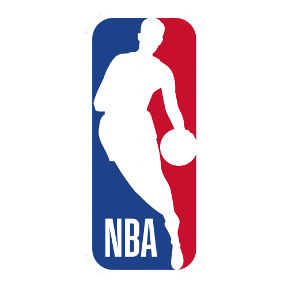
NBA SCOREBOARD
SUNDAY'S GAMES
Top Headlines
- Sources: Mets land Soto on 15-year, $765M deal
- Chiefs clinch AFC West on another walk-off FG
- Tide AD to reevaluate scheduling after CFP snub
- Source: Conforto to join Dodgers for 1 year, $17M
- LeBron (sore foot) sits for first time this season
- Eagles air frustrations as passing attack struggles
- Sources: Ionescu has UCL procedure in thumb
- QB Herbstreit, son of Kirk, commits to Michigan
- Score picks for CFB postseason games
Customize ESPN
ICYMI
Clemson captures ACC title with walk-off 56-yard FG
Nolan Hauser buries a field goal from 56 yards out to push Clemson over SMU.
Best of ESPN+
ESPN Ranking top NFL head coach candidates for 2025 openings
Could Detroit lose both coordinators to head coach jobs? Will Bill Belichick get interest? We stacked the 10 hottest coaching candidates right now.
Jim McIsaac/Getty Images How much Juan Soto would impact Mets, Yankees, more suitors
Juan Soto would make any team better. But exactly by how much better varies. We put a number on it.
ESPN Best NFL players at 102 different skills: Top QBs, WRs, CBs
Most instinctive QB? Most elusive rusher? Top run-stopper? Best ball hawk? We named the NFL's standout in 102 specific skills.
Bill Streicher-Imagn Images 2024-25 NBA rookie rankings: Jared McCain, Dalton Knecht, more
Six weeks into the season, there's a 76ers rookie atop the rankings. Here's our list and what scouts are saying about a Thunder rookie and this class thus far.
Trending Now
Melissa Tamez/Getty Images Inside Lonzo Ball's unprecedented 1,000-day return to the NBA
What was originally thought to be a minor injury turned into "search and rescue" procedures and one surgery no NBA player ever had done. This is the untold story of Ball's harrowing road back to the game.
Ian Johnson/Icon Sportswire Final 2024 college football Bottom 10: Stacking up best of worst
From winless Kent State to woeful Ryan Day, the final votes are in for the list no one wants to make.
Illustration by ESPN The dominance of young Derrick Henry
Before he became a generational star, Derrick Henry was trying to convince the world he could play running back.
V Week 2024
ESPN V Foundation
The V Foundation continues the fight against cancer. If you're able to, help us defeat cancer by donating at v.org/donate.
NFL Playoff Machine
Mark J. Rebilas/USA TODAY Sports Simulate playoff matchups
Predict playoff pairings by selecting the winners of the remaining regular-season games to generate potential scenarios.
ESPN+ on Disney+
Mike Windle/Getty Images for ESPN How to watch ESPN+ on Disney+: Frequently asked questions
Starting Dec. 4, ESPN+ will be available on Disney+. Check out how to watch NHL, MLB, Pat McAfee, 30 for 30s and other exciting events now.
Get a custom ESPN experience
Enjoy the benefits of a personalized account
Select your favorite leagues, teams and players and get the latest scores, news and updates that matter most to you.